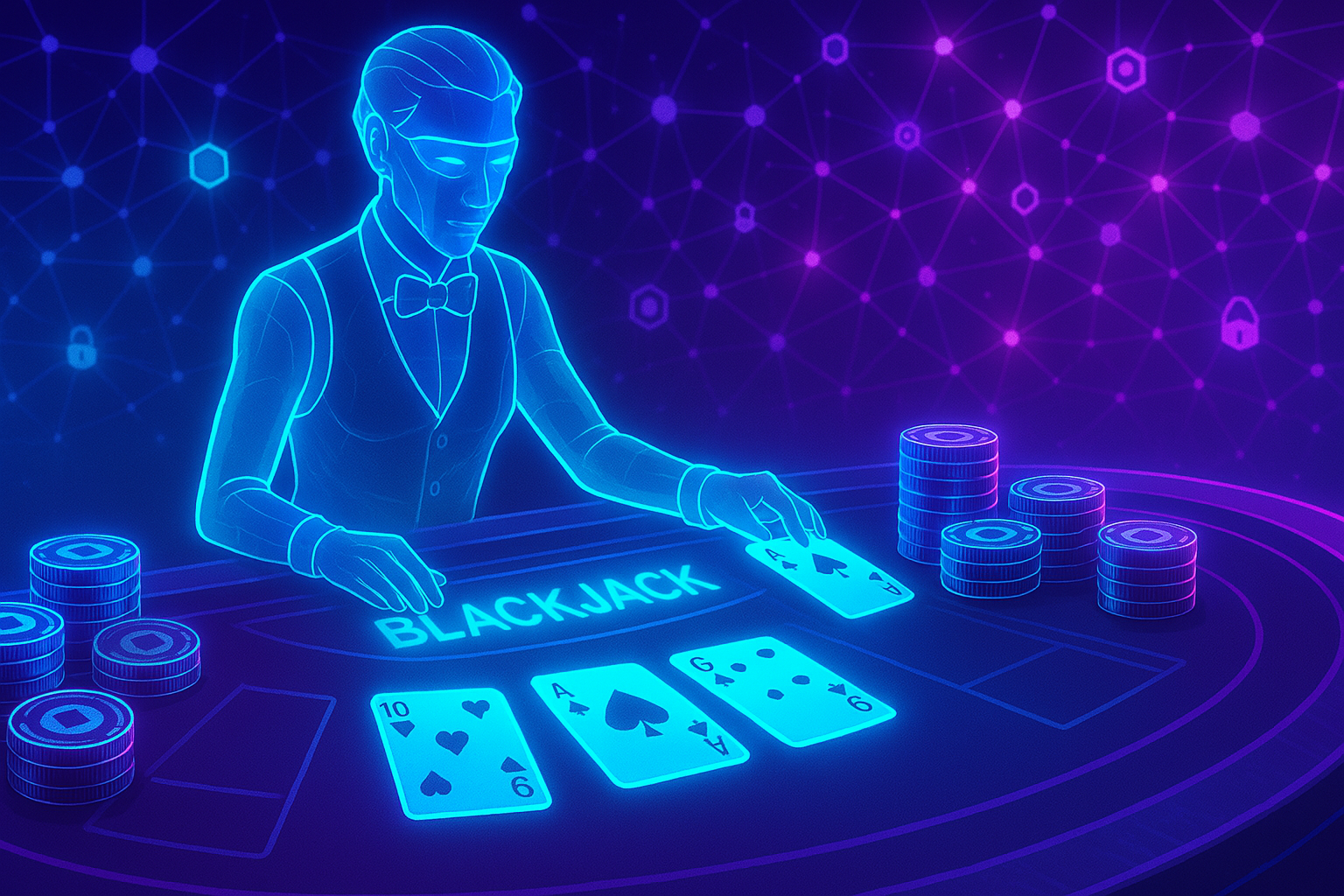
1. Understanding Ryo Currency and Decentralization
Ryo Currency is derived from Monero, emphasizing privacy and secure transactions. Because it currently lacks traditional smart contract support, integrating Ryo involves interacting with the blockchain through wallet RPCs and full-node APIs. In our decentralized blackjack game, Ryo is used for placing bets and handling payouts without relying on a central authority.
Decentralization Goals:
- Trustless Betting: Ensure that players can wager using Ryo in a transparent and secure manner.
- Distributed Game Logic: Avoid a single point of failure by running the game logic on a peer-to-peer network.
- Provably Fair Randomness: Use cryptographic commit–reveal schemes or verifiable random functions (VRFs) to ensure that card shuffling and dealing are fair.
2. Game Architecture and Mechanics
Game Mechanics
Blackjack Rules: Define standard blackjack rules (deck count, dealer behavior, payout rules) and incorporate Ryo transactions for betting.
Provably Fair Dealing: Use a commit–reveal protocol to generate a verifiable random order for cards. Each participant can commit to a random seed (via a cryptographic hash), then reveal it later to ensure fairness.
Decentralized Architecture
Peer-to-Peer (P2P) Game Logic: Utilize decentralized frameworks to share game state and logic across nodes.
On-Chain Settlement: While the game logic runs off-chain, use the blockchain for final bet settlement via Ryo transactions.
3. Blockchain Integration with Ryo Currency
Since Ryo is built on Monero’s architecture, most interactions occur through RPC calls. This is essential for monitoring transactions, handling bets, and processing payouts.
Key Resources:
- Ryo Currency WALLET-RPC CALLS – This is a list of the ryo-wallet-rpc calls, their inputs and outputs, and examples of each.
- Ryo Currency DAEMON-RPC CALLS –This is a list of the ryo-daemon-rpc calls, their inputs and outputs, and examples of each.
- Monero JSON-RPC API Docs – Learn how to interact with the wallet RPC for sending/receiving transactions.
- monero-javascript – A JavaScript library to simplify Ryo (and Monero) wallet integration, allowing your application to manage wallet connections and transactions seamlessly.
- Monero Wallet RPC Boilerplate – A boilerplate project for interacting with the Monero/Ryo network via Node.js.
4. Backend Development
The backend is crucial for managing game sessions, processing Ryo transactions, and coordinating real-time interactions between players.
Framework Options:
- Node.js: Use Express.js or Fastify to create a lightweight server that integrates with Ryo’s RPC API.
- Express WebSocket Starter – For real-time communications.
- Python: Frameworks like Flask or FastAPI can be used if you prefer Python’s ecosystem for handling cryptographic operations and game logic.
- Rust: For a more security-focused approach, consider Rust frameworks like Rocket or Axum, which are ideal if you plan to develop trustless modules.
5. Frontend Development
The user interface (UI) enables players to connect their Ryo wallets, place bets, and play blackjack in real time.
Framework Options:
- React.js: Build a modern, interactive UI. Consider pairing it with Tailwind CSS for rapid styling.
- Vue.js: Lightweight and flexible, Vue can be an excellent choice.
- Vue WebSockets Example – Facilitates real-time communication.
- Svelte: Offers ultra-fast UI updates and a streamlined development process.
Game UI Starter Templates:
- Phaser.js Blackjack Demo – A demo that can be adapted to include Ryo-based betting logic.
- Monero Wallet Web UI – Although designed for Monero, it can be modified for Ryo integration, helping users connect their wallets and manage transactions.
6. Implementing Decentralized Fairness and P2P Communication
To ensure that the game is provably fair, implement cryptographic techniques for random number generation and utilize decentralized networking protocols.
Cryptographic Fairness:
Commit–Reveal Protocol: Each player commits to a hash of a random seed before the game, then reveals the seed after commitments are locked in. This method ensures that no single party controls the randomness.
P2P Networking:
- Libp2p WebRTC Example – A modular network stack that facilitates decentralized communication between nodes.
- WebRTC: Enables real-time, peer-to-peer communication directly between browsers.
7. Deployment and Hosting
For a truly decentralized application, host your frontend on decentralized platforms and deploy your backend in a scalable, secure environment.
Hosting Options:
- IPFS (InterPlanetary File System) – Deploy your static assets (frontend code) on IPFS for decentralized hosting.
- Docker and VPS: Containerize your backend services for easy deployment and scalability using Docker and host them on a VPS or cloud service.
8. Bringing It All Together
Development Roadmap:
- Prototype the Game Logic: Start by building a basic blackjack game using a framework like Phaser.js. Adapt the demo for your specific game rules and fairness requirements.
- Integrate Ryo Transactions: Use monero-javascript and the Ryo Currency Wallet RPC Calls / Monero Wallet RPC Boilerplate to integrate Ryo for placing bets and processing payouts.
- Establish Decentralized Communication: Set up a P2P network using Libp2p and implement a commit–reveal scheme to ensure provably fair card shuffling.
- Build and Connect the Frontend: Develop an engaging user interface using React.js, Vue.js, or Svelte, and integrate real-time updates with WebSockets.
- Deploy on Decentralized Platforms: Host your frontend on IPFS and deploy your backend using Docker to maintain a decentralized architecture.
Open-Source and Community Engagement:
- Documentation: Create detailed developer and user documentation to encourage community contributions.
- GitHub Repository: Host your code on GitHub under an open-source license (e.g., MIT, GPL) to foster collaboration.
9. Skills Needed to Develop This Game
To successfully build a decentralized blackjack game, you’ll need to learn the following skills:
- Programming Fundamentals: JavaScript, Python, Rust (optional).
- Web Development: React.js or Vue.js, Node.js, WebSockets.
- Game Development: Phaser.js or Godot.
- Blockchain Integration: Ryo Wallet RPC, smart contracts.
- Decentralized Networking: WebRTC, Libp2p.
- Security & Fairness: Commit-reveal cryptographic protocols.
Conclusion
Developing a decentralized blackjack game with Ryo Currency involves integrating blockchain transactions, implementing fair gaming logic, and leveraging modern web development frameworks. By using established starter templates and frameworks, you can accelerate development while ensuring security and decentralization.
For further exploration:
- Ryo Currency WALLET-RPC CALLS
- Ryo Currency DAEMON-RPC CALLS
- Monero JSON-RPC API Docs
- monero-javascript
- Monero Wallet RPC Boilerplate
- Express WebSocket Starter
- Phaser Blackjack Demo
- Libp2p WebRTC Example
- IPFS Web Hosting Guide
By following these guidelines and utilizing the provided resources, you can create a decentralized, open-source blackjack game that leverages the security and privacy of Ryo Currency while providing a fair and engaging gaming experience.